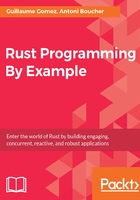
Slices
Arrays are fixed-size, but if we want to create a function that works with arrays of any size, we need to use another type: a slice.
A slice is a view into a contiguous sequence: it can be a view of the whole array, or a part of it. Slices are fat pointers, in addition to the pointer to the data, they contain a size. Here's a function that returns a reference to the first element of a slice:
fn first<T>(slice: &[T]) -> &T { &slice[0] }
Here, we use a generic type without bound since we don't use any operation on values of the T type. The &[T] parameter type is a slice of T. The return type is &T, which is a reference on values of the T type. The body of the function is &slice[0], which returns a reference to the first element of the slice. Here's how to call this function with an array:
println!("{}", first(&array));
We can create slice for only a portion of an array, as shown in the following example:
println!("{}", first(&array[2..]));
&array[2..] creates a slice that starts at the 2 index until the end of the array (hence no index after ..). Both indices are optional, so we could also write &array[..10] for the first 10 elements of the array, &array[5..10] for the elements with the 5 to 9 index (inclusive), or &array[..] for all the elements.