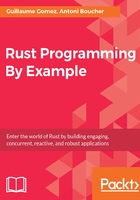
Arrays
An array is a fixed-size collection of elements of the same type. We declare them with square brackets:
let array = [1, 2, 3, 4]; let array: [i16; 4] = [1, 2, 3, 4];
The second line shows how to specify the type of an array. An alternative way to do that is to use a literal suffix:
let array = [1u8, 2, 3, 4];
A literal suffix is the composition of a literal (that is, a constant) and a type suffix, so with the 1 constant and the u8 type, we get 1u8. Literal suffixes can only be used on numbers. This declares an array of 4 elements of the u8 type. Array indexing starts at 0 and bounds checking is done at runtime. Bounds checking is used to prevent accessing memory that is out of bounds, for instance, trying to access the element after the end of an array. While this can slow down the software a bit, it can be optimized in many cases. The following code will trigger a panic because the 4 index is one past the end of the array:
println!("{}", array[4]);
At runtime, we see the following message:
thread 'main' panicked at 'index out of bounds: the len is 4 but the index is 4', src/main.rs:5:20 note: Run with `RUST_BACKTRACE=1` for a backtrace.
Another way to declare an array is:
let array = [0u8; 100];
This declares an array of 100 elements, where all of them are 0.