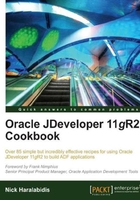
Using a custom property to populate a sequence attribute
In this recipe, we will go over a generic programming technique that you can use to assign database sequence values to specific entity object attributes. Generic functionality is achieved by using custom properties. Custom properties allow you to define custom metadata that can be accessed by the ADF business components at runtime.
Getting ready
We will add this generic functionality to the custom entity framework class. This class was created back in the Setting up BC base classes recipe in Chapter 1, Pre-requisites to Success: ADF Project Setup and Foundations. The custom framework classes in this case reside in the SharedComponets
workspace. This workspace was created in the recipe Breaking up the application in multiple workspaces, Chapter1, Pre-requisites to Success: ADF Project Setup and Foundations. You will need to create a database connection to the HR
schema, if you are planning to run the recipe's test case. You can do this either by creating the database connection in the Resource Palette and dragging-and-dropping it to Application Resources | Connections, or by creating it directly in Application Resources | Connections.
How to do it...
- Start by opening the
SharedComponets
workspace in JDeveloper. If needed, follow the steps in the referenced recipe to create it. - Locate the custom entity framework class in the
SharedBC
project and open it in the editor. - Click on the Override Methods… icon on the toolbar (the green left arrow) to bring up the Override Methods dialog.
- From the list of methods that are presented, select the create() method and click OK. JDeveloper will insert a
create()
method in to the body of your custom entity class. - Add the following code to the
create()
method immediately after the call tosuper.create()
:// iterate all entity attributes for (AttributeDef atrbDef : this.getEntityDef().getAttributeDefs()) { // check for a custom property called CREATESEQ_PROPERTY String sequenceName = (String)atrbDef.getProperty(CREATESEQ_PROPERTY); if (sequenceName != null) { // create the sequence based on the custom property sequence name SequenceImpl sequence = new SequenceImpl(sequenceName, this.getDBTransaction()); // populate the attribute with the next sequence number this.populateAttributeAsChanged(atrbDef.getIndex(),sequence.getSequenceNumber()); } }
How it works...
In the previous code, we have overridden the create()
method for the custom entity framework class. This method is called by the ADF framework each time a new entity object is constructed. We call super.create()
to allow the framework processing, and then we retrieve the entity's attribute definitions by calling getEntityDef().getAttributeDefs()
. We then iterate over them, calling getProperty()
for each attribute definition. getProperty()
accepts the name of a custom property defined for the specific attribute. In our case, the custom property is called CreateSequence
and it is indicated by the constant definition CREATESEQ_PROPERTY
, representing the name of the database sequence used to assign values to the particular attribute. Next, we instantiate a SequenceImpl
object using the database sequence name retrieved from the custom property. Note that this does not create the database sequence, rather an oracle.jbo.server.SequenceImpl
object representing a database sequence.
Finally, the attribute is populated with the value returned from the sequence—via the getSequenceNumber()
call—by calling populateAttributeAsChanged()
. This method will populate the attribute without marking the entity as changed. By calling populateAttributeAsChanged()
, we will avoid any programmatic or declarative validations on the attribute while marking the attribute as changed, so that its value is posted during the entity object DML. Since all of the entity objects are derived from the custom entity framework class, all object creations will go through this create()
implementation.
There's more...
So how do you use this technique to populate your sequence attributes? First you must deploy the SharedComponets
workspace into an ADF Library JAR and add the library to the project where it will be used. Then, you must add the CreateSequence
custom property to the specific attributes of your entity objects that need to be populated by a database sequence. To add a custom property to an entity object attribute, select the specific attribute in the entity Attributes tab and click on the arrow next to the Add Custom Property icon (the green plus sign) in the Custom Properties tab. From the context menu, select Non-translatable Property .

Click on the Property field and enter CreateSequence
. For the Value enter the database sequence name that will be used to assign values to the specific attribute. For the Employee entity object example mentioned earlier, we will use the EMPLOYEES_SEQ database sequence to assign values to the EmployeeId attribute.
See also
- Breaking up the application in multiple workspaces, Chapter 1, Pre-requisites to Success: ADF Project Setup and Foundations
- Setting up BC base classes, Chapter 1, Pre-requisites to Success: ADF Project Setup and Foundations
- Overriding doDML() to populate an attribute with a gapless sequence, in this chapter
- Creating and applying property sets, in this chapter