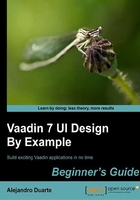
Time for action – separating business classes from UI classes
Vaadin is mostly about UI. Vaadin's motto thinking of U and I is not in vain. I said mostly because you can additionally find a bunch of useful add-ons to deal with server-side technologies. Even Vaadin itself ships with some data components.
Note
There are lots of integration add-ons for Vaadin. For instance, you can integrate with technologies such as Groovy, Spring Framework, SQL, JPA, Hibernate, Lucene, Google App Engine Datastore, Quartz, JasperReports, JFreeChart, OpenLayers, Google Maps, OpenID, and even Twitter! All this with one hand tied behind your back. Visit https://vaadin.com/directory.
Most, if not all, applications have some kind of business logic. That is, something to do with the application's data. If you are an experienced developer you will agree with me that separating business logic from UI logic makes a lot of sense. For the "Time It" application, we will use two Java packages to separate business logic from UI logic:
- Create a new Vaadin project named time-it using your IDE.
- Delete the all the generated classes. We will add our own classes in a minute.
- Create the following package. Don't worry about the classes right now. Just create the Java packages
timeit
,timeit.biz
,timeit.biz.test
, andtimeit.ui
: - Browse the book's source code and copy all the classes inside the
biz
package and paste them into your ownbiz
package. Don't copyTimeItUI.java
, we will work on that through the chapter. - Create a new class with the
TimeItUI
name inside theui
package. - Update your
web.xml
file to useTimeItUI
as the starting application:<servlet> <servlet-name>Time It</servlet-name> <servlet-class>com.vaadin.server.VaadinServlet</servlet-class> <init-param> <description> Vaadin UI class to use</description> <param-name>UI</param-name> <param-value>timeit.ui.TimeItUI</param-value> </init-param> </servlet> <servlet-mapping> <servlet-name>Time It</servlet-name> <url-pattern>/*</url-pattern> </servlet-mapping>
- Let's start with an outline of our
TimeItUI
class. Modify yourTimeItUI
class to match something like this:public class TimeItUI extends UI { ... some variables ... @Override protected void init(VaadinRequest request) { ... } private void initCombo() { ... } private void initButton() { ... } private void initLayout() { ... } public boolean validate() { ... } public void runSelectedTest() { ... } private void showResults(Collection<String> results) { ... } }
What just happened?
Of course, the TimeItUI
class won't compile. But now you have an outline with the structure of methods we will implement through the chapter. Just keep reading.
Most of the application is about business (the biz
package) with only one class handling our UI logic. However, we will focus on the UI part. Business classes are not puzzling at all. We will use them as black boxes in our TimeItUI
class (that's the reason we are just copying them).
Tip
I've faced several situations where I needed to modify UI components calling lots of business methods on classes that were completely unknown to me. Sometimes, I only had access to remote EJBs' interfaces code, there was no way to look at the actual implementation. When modifying UI layers, treat business logic as black boxes and use unit testing to prevent introducing regression bugs.
UI components as class members
In the example of the previous chapter, we created UI components as they were needed inside methods. This time, for the "Time It" application, we will declare most of the UI components as class members.