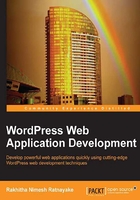
Planning the portfolio application tables
As described in this book, the portfolio management system will make use of the existing tables in every possible scenario. However, it's hard to imagine even an average web application without using custom tables. So, here we are going to identify the possible custom tables for our system. You might need to refer back to the planning section of Chapter 1, WordPress As a Web Application Framework, in order to recollect the system requirements. We planned to create a functionality to allow subscribers to follow developers in the system. Let's discuss the requirements in detail to identify the potential tables.
Developers can build their portfolios with personal info, services, projects, articles, or any other necessary things to demonstrate their skills. Each user will have their own RSS feed containing all the activities within the system. Followers will be allowed to subscribe to multiple developers.
This is a very simple and practical scenario for identifying the use of custom tables. We can easily scale this up so that it is compatible with the complex systems. Developers are stored as users of the system. Therefore, we only have the choice of the wp_usermeta
table for additional features. It's highly impractical to keep user activities in the wp_usermeta
table. So, we need to create our first custom table named user_activities
to implement this feature.
Types of tables in web applications
Database tables of web applications can be roughly categorized into three sections, namely:
- Master tables: These tables contain predefined or configuration data for the application that rarely gets changed. The wp_options table can be considered the perfect example for this type of table in the WordPress context.
- Application data tables: These tables contain the highly dynamic core application data. wp_users can be considered good examples for these types of tables in the WordPress context.
- Transaction tables: These tables contain the highest volume of data in any application. Records in these tables rarely get changed, but new records will be added at a faster rate. It's difficult to find good examples for these types of tables in the WordPress context.
Based on the categories, we can clearly see that the user_activities
table falls into the transaction table category. Next, we need to allow the followers to subscribe to developers. So, we need another transaction type table named subscribed_developers
. We can assume that most of the transaction type tables will need their own custom tables. For now, we are going to stick with these two tables and additional custom tables will be added in later chapters when needed.
Creating custom tables
In typical scenarios, we create the database tables manually before moving into the implementation. With the WordPress plugin-based architecture, it's certain that we might need to create custom tables using plugins in the later stages of the projects. Creating custom tables through plugins involves certain predefined procedures recommended by WordPress. Since table creation is a one-time task, we can implement the process on plugin activation. So, let's get started by creating a new folder named wpwa_database_manager
inside the /wp-content/plugins
folder.
Then, create a PHP file inside the folder and save it as wpwa-database-manager.php
. Now it's time to add the plugin definition as shown in the following code:
<?php class WPWA_Database_Manager { public function __construct(){ register_activation_hook( __FILE__, array( $this, 'create_custom_tables' ) ); } public function create_custom_tables() { // Creating Database Tables } }
Once the activation hook is defined inside the plugin constructor, we can implement the create_custom_tables
function to create the necessary tables for our application. Basically, we can execute direct SQL queries using the $wpdb->query
function to create all the tables we need. WordPress recommends using a built-in function called dbDelta
for creating custom tables. This function is located in a file outside the default process and hence we need to load it manually within our plugins. Let's create two tables for our application using the dbDelta
function.
public function create_custom_tables() { global $wpdb; $table_name = $wpdb->prefix.user_activities; require_once( ABSPATH . 'wp-admin/includes/upgrade.php' ); $sql = "CREATE TABLE $table_name ( id mediumint(9) NOT NULL AUTO_INCREMENT, time datetime DEFAULT '0000-00-00 00:00:00' NOT NULL, user_id mediumint(9) NOT NULL, activity text NOT NULL, url VARCHAR(255) DEFAULT '' NOT NULL, UNIQUE KEY id (id) );"; dbDelta($sql); // subscribed_developers will be created in a similar manner }
Initially, we have to include the upgrade.php
file to make use of the dbDelta
function. The next most important thing is to use the prefix for database tables. By default, WordPress creates a prefix named wp_
for all the tables. It's important to use the existing prefix to keep consistency and avoid issues in multisite scenarios. Finally, you can define your table creation query and use the dBDelta
function to implement it on the database.
Note
Check out the guidelines at http://codex.wordpress.org/Creating_Tables_with_Plugins for creating the table creation query as the dbDelta
function can be tricky in certain scenarios.
We created the custom tables using the dbDelta
function inside plugin activation. WordPress recommends the dbDelta
function over direct SQL queries for table creation since it examines the current table structure, compares it with the desired table structure, and makes the necessary modifications without breaking the existing database tables. Apart from table creation, we can execute quite a few database-related tasks on plugin activation, such as altering tables, populating initial data to custom tables, and upgrading the plugin tables.
We looked at the necessity of custom tables for web applications. Even though custom tables offer you more flexibility within WordPress, there will be a considerable amount of limitations, as listed in the following section:
- They are difficult to manage in WordPress upgrades.
- WordPress default backups will not include custom tables.
- No built-in functions for accessing databases. All the queries, filtering, and validation need to be done from scratch using the existing
$wpdb
variable. - User interfaces for displaying the data of these tables need to be created from scratch.
Therefore, you should avoid creating custom tables in all possible scenarios unless you have a distinct advantage from the perspective of your application.
Tip
The WordPress PODS framework works very well in managing custom post types with custom tables. You can have a look at the source code at http://wordpress.org/plugins/pods/ for learning the use of custom tables.
Detailed exploration about the PODS framework will be provided in the next chapter, Chapter 4, The Building Blocks of Web Applications.