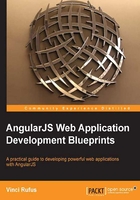
Yeoman – the workflow tool
Yeoman prefers to be known as a workflow rather than just a tool. It is actually a collection of three tools that help you manage your workflow efficiently. The tools that come as a part of Yeoman are as follows:
- Yo: This is a scaffolding tool and using the numerous generators available, one can quickly create the skeleton of your project. Yo has a generator to build AngularJS apps and we will be using that later in this chapter.
- Grunt: This is used to run the tasks that will help you preview, test, and build the app.
- Bower: This is an ideal tool for dependency management. Yeoman uses it to automatically search and download the necessary scripts.
Let's go about installing Yeoman and playing around with it a bit.
Installing Yeoman
To install Yeoman, make sure you are running it with administrative privileges. Enter the following command in the terminal:
sudo npm install –g yo
Next, let's install the AngularJS generator using the following command:
sudo npm install - g generator-angular
Now, let's create our project directory and create the skeleton for our project. We will call our app Yoho; so, first let's create a folder called yoho
. Enter the following command in the terminal:
mkdir yoho cd yoho yo angular
It's now going to start asking a series of questions, answer Y
for all except the question, "Would you like to use Sass (with Compass)?". Answer N
for this one.
Note
The reason we say no here is because for now we will use vanilla CSS. Using Saas and Compass is however strongly recommended while building large applications.
Once yo-angular
has finished doing whatever it had to do, go into your yoho
folder and you'll notice a whole bunch of files and folders, as shown in the following screenshot:

Yeoman has created the skeleton of your AngularJS app along with everything you will need for this project.
Before we go into the details of the different files, one thing to note is that your node_modules
folder is empty. This means Yeoman has only created the package.json
file with all devdependencies
listed out, but hasn't downloaded them yet.
We will need to run the following command:
npm install
This will download and install all the dependencies listed out in the package.json
file. Once it's finished installing, verify that the node_modules
folder now has folders such as grunt-contrib-clean
and grunt-contrib-concat
within it.
Ok, now, let's try and make sense of all the files that Yeoman has created. Refer to the following table:

Let's install our Node.js modules by running the following command in the terminal:
npm install
Running your app
Earlier in this chapter, we saw how to set up a server using Node.js and ExpressJS. Yeoman comes with its own server and running it is as simple as running the following command in your terminal:
grunt serve
Tip
The grunt server
command is deprecated although it might still work for some.
This will open up a new browser window and will show you the default welcome screen, as shown in the following screenshot:

If you recollect looking at the main.js
file (under scripts/controllers
) and the main.html
file (under views
), you'll notice the page that is being rendered. Let's play around a bit.
Open the scripts/controllers/main.js
file and you'll find a controller called MainCtrl
and a model called awesomeThings
. Let's add some more items to this array as follows:
'use strict'; angular.module('yohoApp') .controller('MainCtrl', function ($scope) { $scope.awesomeThings = [ 'HTML5 Boilerplate', 'AngularJS', 'Karma', 'E2E', 'Protractor' ]; });
Let's display our awesomeThings
array in the view. Please add the following code to the main.html
file as follows:
<ul class="row"> <li ng-repeat="things in awesomeThings">{{things}}</li> </ul>
Save the file and switch to the browser. VOILA! The page updated on its own, you didn't have to reload the page in the browser.
The browser updates the moment you save your file, no more hitting the refresh button. Isn't that a big relief and a productivity boost!
This works thanks to a nifty module called LiveReload
. You'll find this being installed as a part of devDependencies
in the package.json
file. You'll also notice Grunt tasks for it created in your gruntfile.js
file.
So now you can have the server running and place your browser window and your text editor arranged side-by-side, and watch your app update as you write your code and save the file.