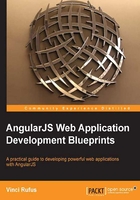
Setting up Node.js
Depending on your technology stack, I strongly recommend you have either Ruby or Node.js installed.
In case of AngularJS, most of the productivity tools or plugins are available as Node Package Manager (npm), and, hence, we will be setting up Node.js along with npm. Node.js is an open source JavaScript-based platform that uses an event-based Input/output model, making it lightweight and fast.
Let us head over to www.nodejs.org and install Node.js. Choose the right version as per your operating system.
The current version of Node.js at the time of writing this book is v0.10.x which comes with npm built in, making it a breeze to set up Node.js and npm.
Tip
Node.js doesn't come with a Graphical User Interface (GUI), so to use Node.js, you will need to open up your terminal and start firing some commands. Now would also be a good time to brush up on your DOS and Unix/Linux commands.
After installing Node.js, the first thing you'd want to check is to see if Node.js has been installed correctly.
So, let us open up the terminal and write the following command:
node –-version
This should output the version number of Node.js that's installed on your system. The next would be to see what version of npm we have installed. The command for that would be as follows:
npm –-version
This will tell you the version number for your npm.
Creating a simple Node.js web server with ExpressJS
For basic, simple AngularJS apps, you don't really need a web server. You can simply open the HTML files from your filesystem and they would work just fine. However, as you start building complex applications where you are passing data in JSON, web services, or using a Content Delivery Network (CDN), you would find the need to use a web server.
The good thing about AngularJS apps is that they could work within any web server, so if you already have IIS, Apache, Nginx, or any other web server running on your development environment, you can simply run your AngularJS project from within the web root folder.
In case you don't have a web server and are looking for a lightweight web server, then let us set one up using Node.js and ExpressJS.
One could write the entire web server in pure Node.js; however, ExpressJS provides a nice layer of abstraction on top of Node.js so that you can just work with the ExpressJS APIs and don't have to worry about the low-level calls.
So, let's first install the ExpressJS module for Node.js.
Open up your terminal and fire the following command:
npm install -g express-generator
This will globally install ExpressJS. Omit the –g
to install ExpressJS locally in the current folder.
When installing ExpressJS globally on Linux or Mac, you will need to run it via sudo
as follows:
sudo npm install –g express-generator
This will let npm
have the necessary permissions to write to the protected local folder under the user. The next step is to create an ExpressJS app; let us call it my-server
. Type the following command in the terminal and hit enter:
express my-server
You'll see something like this:
create : my-server create : my-server/package.json create : my-server/app.js create : my-server/public create : my-server/public/javascripts create : my-server/public/images create : my-server/public/stylesheets create : my-server/public/stylesheets/style.css create : my-server/routes create : my-server/routes/index.js create : my-server/routes/user.js create : my-server/views create : my-server/views/layout.jade create : my-server/views/index.jade install dependencies: $ cd my-server && npm install run the app: $ DEBUG=my-server ./bin/www
This will create a folder called my-server
and put in a bunch of files inside the folder.
Note
The package.json
file is created, which contains the skeleton of your app. Open it and ensure the name says my-server
; also, note the dependencies listed.
Now, to install ExpressJS along with the dependencies, first change into the my-server
directory and run the following command in the terminal:
cd my-server npm install
Now, in the terminal, type in the following command:
npm start
Open your browser and type http://localhost:3000
in the address bar. You'll get a nice ExpressJS welcome message. Now to test our Address Book App created in Chapter 1, Introduction to AngularJS and the Single Page Application, we will copy our index.html
, scripts.js
, and styles.css
into the public
folder located within my-server
.
Note
I'm not copying the angular.js
file because we'll use the CDN version of the AngularJS library.
Open up the index.html
file and replace the following code:
<script src= "angular.min.js" type="text/javascript"> </script>
With the CDN version of AngularJS as follows:
<script src="//ajax.googleapis.com/ajax/libs/angularjs/1.2.17/angular.min.js"></script>
A question might arise, as to what if the CDN is unreachable. In such cases, we can add a fall back to use a local version of the AngularJS library.
We do this by adding the following script after the CDN link is called:
<script>window.angular || document.write('<script src="lib/angular/angular.min.js"><\/script>');</script>
Save the file in the browser and enter localhost:3000/index.html
. Your Address Book is now running from a server and taking advantage of Google's CDN to serve the AngularJS file.
Tip
Referencing the files using only //
is also called the protocol independent absolute path. This means that the files are requested using the same protocol that is being used to call the parent page. For example, if the page you are loading is via https://
, then the CDN link will also be called via HTTPS.
This also means that when using //
instead of http://
during development, you will need to run your app from within a server instead of a filesystem.