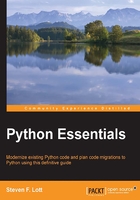
The input() function
For simple applications, the input()
function can be used to gather input from a user. This function writes a prompt and accepts input. The returned value is a string. We might use this in a script file as follows:
c= float(input("Temperature, C: ")) print("f =", 32+9*c/5)
This will write a simple prompt on the console, and accept a string as input. The string value will be converted to a floating-point number, if possible. If the string is not a valid number, the float()
function will raise an exception. This will then print a line of output.
Here's how it looks when we run it:
MacBookPro-SLott:Code slott$ python3 Chapter_4/ex_1.py Temperature, C: 11 f = 51.8
We've highlighted the command, which is entered after the OS shell prompt. The statements in the script file, named as part of the command, are executed in order.
Our input to Python, 11
, is also highlighted, to show how the input()
function supports simple interaction.
The input()
function only returns a Unicode string. Our script is responsible for any further parsing, validation, or conversion.
When working on simple console applications, there are some additional libraries which may prove helpful. There is a getpass
module which helps to get passwords by suppressing the character echo that's a default feature of console input. This is highly recommended as an alternative to plain passwords in a parameter file or the passwords provided on the command line.
We can include the readline
module to provide a comprehensive history of input that makes it easier for interactive users to recover previous inputs. Additionally, the rlcompleter
module can be used to provide auto-complete features so that users only need to enter partial commands.
Beyond this, Python can include an implementation of the Linux curses
library for building richly interactive character user interface (CUI) applications. This is sometimes used to provide colored output on the console, something that can make a complex log easier to read.
Python is used in a wide variety of application contexts. When building a web server, for example, the idea of console or command-line input is utterly out of place. Similarly, the input()
function isn't going to be part of a GUI application.