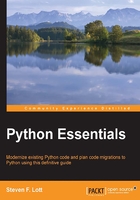
Working with sequences
In this chapter, we'll introduce Python sequence collections. We'll look at strings and tuples as the first two examples of this class. Python offers a number of other sequence collections; we'll look at them in Chapter 6, More Complex Data Types. All of these sequences have common features.
Python sequences identify the inpidual elements by position. Position numbers start with zero. Here's a tuple
collection with five elements:
>>> t=("hello", 3.14, 23, None, True) >>> t[0] 'hello' >>> t[4] True
In addition to the expected ascending numbers, Python also offers reverse numbering. Position -1
is the end of the sequence:
>>> t[-1] True >>> t[-2] >>> t[-5] 'hello'
Note that position 3 (or -2) has a value of None
. The REPL doesn't display the None
object, so the value of t[-2]
appears to be missing. For more visible evidence that this value is None
, use this:
>>> t[3] is None True
The sequences use an extra comparison operator, in
. We can ask if a given value occurs in a collection:
>>> "hello" in t True >>> 2.718 in t False
Slicing and dicing a sequence
We can extract a subsequence, called a slice, from a sequence using more complex subscript expressions. Here's a substring of a longer string:
>>> "multifaceted"[5:10] 'facet'
The [5:10]
expression is a slice which starts at position 5 and extends to the position before 10. Python generally relies on "half-open" intervals. The starting position of a slice is included whereas the stop position is excluded.
We can omit the starting position from a slice, writing [:pos]
. If the start value of a slice is omitted, it's 0. We can omit the ending, also, writing it as [pos:]
. If the stop value of a slice is omitted, it's the length of the sequence, given by the len()
function.
The way that Python uses these half-open intervals means that we can partition a string with very tidy syntax:
>>> "multifaceted"[:5] 'multi' >>> "multifaceted"[5:] 'faceted'
In this example, we've taken the first five characters in the first slice. We've taken everything after the first five characters in the second slice. Since the numbers are both five, we can be completely sure that the entire string is accounted for.
And yes, we can omit both values from the slice: "word"[:]
will create a copy of the entire string. This is an odd but sometimes useful construct for duplicating an object.
There's a third parameter to a slice. We generally call the positions start, stop, and step. The step size is 1 by default. We can use a form such as "abcdefg"[::2]
to provide an explicit step, and pick characters in positions 0, 2, 4, and 6. The form "abcdefg"[1::2]
will pick the odd positions: 1, 3, and 5.
The step size can also be negative. This will enumerate the index values in reverse order. The value of "word"[::-1] is 'drow'
.