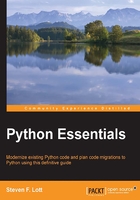
The math libraries
The Python library has six modules relevant to mathematical work. These are described in Chapter 9, Numeric and Mathematical Modules, of the Python Standard Library document. Beyond this, we have external libraries such as NumPy (http://www.numpy.org) and SciPy (http://www.scipy.org). These libraries include vast collections of sophisticated algorithms. For an even more sophisticated toolset, the Anaconda project (https://store.continuum.io/cshop/anaconda/) combines NumPy, SciPy, and 18 more packages.
These are the relevant built-in numeric packages:
numbers
: This module defines the essential numeric abstractions. We rarely need this unless we're going to invent an entirely new kind of number.math
: This module has a large collection of functions. It includes basicsqrt()
, the various trigonometric functions (sine, cosine, and so on) and the various log-related functions. It has functions for working with the internals of floating-point numbers. It also has the gamma function and the error function.cmath
: This module is the complex version of themath
library. We use thecmath
library so that we can seamlessly move betweenfloat
andcomplex
values.decimal
: Import theDecimal
class from this module to work with currency values accurately.fractions
: Import theFraction
class to work with a precise rational fraction value.random
: This module contains the essential random number generator. It has a number of other functions to produce random values in various ranges or with various constraints. For examplerandom.gauss()
produces a Gaussian, normal distribution of floating-point values.
The three main ways of importing from these libraries are as follows:
import random
: We use this when we want to be perfectly explicit about the origin of a name elsewhere in our code. We'll be writing code similar torandom.gauss()
andrandom.randint()
using the module name as an explicit qualifier.from random import gauss, randint
: This introduces two selected names from therandom
module into the global namespace. We can usegauss()
andrandint()
without a qualifying module name.from random import *
: This will introduce all of the available names in therandom
module as globals in our application. This is helpful for exploring and experimenting at the>>>
prompt. This may not be appropriate in a larger program because it can introduce a large number of irrelevant names.
A less-commonly used feature allows us to rename objects brought in via the import
statement. We might want to use from cmath import sqrt as csqrt
to rename the cmath.sqrt()
function to csqrt()
. We have to be careful to avoid ambiguity and confusion when using this import-as
renaming feature.