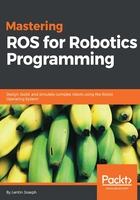
Explaining the xacro model of seven DOF arm
We will define 10 links and 9 joints on this robot and 2 links and 2 joints in the robot gripper.
Let's start by discussing the xacro
definition:
<?xml version="1.0"?> <robot name="seven_dof_arm" xmlns:xacro="http://www.ros.org/wiki/xacro">
Because we are writing a xacro file, we should mention the xacro
namespace to parse the file.
Using constants
We use constants inside this xacro to make robot descriptions shorter and readable. Here, we define the degree to the radian conversion factor, PI value, length, height, and width of each of the links:
<property name="deg_to_rad" value="0.01745329251994329577"/> <property name="M_PI" value="3.14159"/> <property name="elbow_pitch_len" value="0.22" /> <property name="elbow_pitch_width" value="0.04" /> <property name="elbow_pitch_height" value="0.04" />
Using macros
We define macros in this code to avoid repeatability and to make the code shorter. Here are the macros we have used in this code:
<xacro:macro name="inertial_matrix" params="mass"> <inertial> <mass value="${mass}" /> <inertia ixx="1.0" ixy="0.0" ixz="0.0" iyy="0.5" iyz="0.0" izz="1.0" /> </inertial> </xacro:macro>
This is the definition of the inertial matrix
macro in which we can use mass
as its parameter:
<xacro:macro name="transmission_block" params="joint_name"> <transmission name="tran1"> <type>transmission_interface/SimpleTransmission</type> <joint name="${joint_name}"> <hardwareInterface>PositionJointInterface</hardwareInterface> </joint> <actuator name="motor1"> <hardwareInterface>PositionJointInterface</hardwareInterface> <mechanicalReduction>1</mechanicalReduction> </actuator> </transmission> </xacro:macro>
In the section of the code, we can see the definition using the transmission
tag.
The transmission
tag relates a joint to an actuator. It defines the type of transmission that we are using in a particular joint and the type of motor and its parameters. It also defines the type of hardware interface we use when we interface with the ROS controllers.
Including other xacro files
We can extend the capabilities of the robot xacro by including the xacro definition of sensors using the xacro:include
tag. The following code snippet shows how to include a sensor definition in the robot xacro:
<xacro:include filename="$(find mastering_ros_robot_description_pkg)/urdf/sensors/xtion_pro_live.urdf.xacro"/>
Here, we include a xacro definition of sensor called Asus Xtion pro, and this will be expanded when the xacro file is parsed.
Using "$(find mastering_ros_robot_description_pkg)/urdf/sensors/xtion_pro_live.urdf.xacro"
, we can access the xacro definition of the sensor, where find
is to locate the current package mastering_ros_robot_description_pkg
.
We will discuss more on vision processing in Chapter 9, Building and Interfacing Differential Drive Mobile Robot Hardware in ROS.
Using meshes in the link
We can insert a primitive shape to a link or we can insert a mesh file using the mesh
tag. The following example shows how to insert a mesh of the vision sensor:
<visual> <origin xyz="0 0 0" rpy="0 0 0"/> <geometry> <mesh filename="package://mastering_ros_robot_description_pkg/meshes/sensors/xtion_pro_live/xtion_pro_live.dae"/> </geometry> <material name="DarkGrey"/> </visual>
Working with the robot gripper
The gripper of the robot is designed for the picking and placing of blocks and the gripper is on the simple linkage category. There are two joints for the gripper and each joint is prismatic. Here is the joint
definition of one gripper joint:
<joint name="finger_joint1" type="prismatic"> <parent link="gripper_roll_link"/> <child link="gripper_finger_link1"/> <origin xyz="0.0 0 0" /> <axis xyz="0 1 0" /> <limit effort="100" lower="0" upper="0.03" velocity="1.0"/> <safety_controller k_position="20" k_velocity="20" soft_lower_limit="${-0.15 }" soft_upper_limit="${ 0.0 }"/> <dynamics damping="50" friction="1"/> </joint>
Here, the first gripper joint is formed by gripper_roll_link
and gripper_finger_link1
, and the second joint is formed by gripper_roll_link
and gripper_finger_link2
.
The following graph shows how the gripper joints are connected in gripper_roll_link
:

Figure 9 : Graph of the end effector section of seven dof arm robot
Viewing the seven DOF arm in RViz
After discussing the robot model, we can view the designed xacro file in RViz and control each joint using the joint state publisher
node and publish the robot state using the Robot State Publisher
.
The preceding task can be performed using a launch file called view_arm.launch
, which is inside the launch
folder of this package:
<launch> <arg name="model" /> <!-- Parsing xacro and loading robot_description parameter --> <param name="robot_description" command="$(find xacro)/xacro.py $(find mastering_ros_robot_description_pkg)/urdf/ seven_dof_arm.xacro " /> <!-- Setting gui parameter to true for display joint slider, for getting joint control --> <param name="use_gui" value="true"/> <!-- Starting Joint state publisher node which will publish the joint values --> <node name="joint_state_publisher" pkg="joint_state_publisher" type="joint_state_publisher" /> <!-- Starting robot state publish which will publish current robot joint states using tf --> <node name="robot_state_publisher" pkg="robot_state_publisher" type="state_publisher" /> <!-- Launch visualization in rviz --> <node name="rviz" pkg="rviz" type="rviz" args="-d $(find mastering_ros_robot_description_pkg)/urdf.rviz" required="true" /> </launch>
Create the following launch file inside the launch
folder and build the package using the catkin_make
command. Launch the urdf
using the following command:
$ roslaunch mastering_ros_robot_description_pkg view_arm.launch
The robot will be displayed on RViz with the joint state publisher GUI.

Figure 10 : Seven dof arm in RViz with joint_state_publisher
We can interact with the joint slider and move the joints of the robot. We can next discuss what the joint state publisher
is.
Understanding joint state publisher
Joint state publisher is one of the ROS packages that is commonly used to interact with each joint of the robot. The package contains the joint_state_publisher
node, which will find the nonfixed joints from the URDF model and publish the joint state values of each joint in the sensor_msgs/JointState
message format.
In the preceding launch file, view_arm.launch
, we started the joint_state_publisher
node and set a parameter called use_gui
to true as follows:
<param name="use_gui" value="true"/> <!-- Starting Joint state publisher node which will publish the joint values --> <node name="joint_state_publisher" pkg="joint_state_publisher" type="joint_state_publisher" />
If we set use_gui
to true, the joint_state_publisher
node displays a slider based control window to control each joint. The lower and upper value of a joint will be taken from the lower and upper values associated with the limit
tag used inside the joint
tag. The preceding screenshot shows RViz along with GUI to publish joint states with the use_gui
parameter set to true
.
We can find more on the joint state publisher
package at http://wiki.ros.org/joint_state_publisher.
Understanding the robot state publisher
The robot state publisher
package helps to publish the state of the robot to tf
. This package subscribes to joint states of the robot and publishes the 3D pose of each link using the kinematic representation from the URDF model. We can use the robot state publisher
node using the following line inside the launch file:
<!-- Starting robot state publish which will publish tf --> <node name="robot_state_publisher" pkg="robot_state_publisher" type="state_publisher" />
In the preceding launch file, view_arm.launch
, we started this node to publish the tf
of the arm. We can visualize the transformation of the robot by clicking on the tf
option on RViz shown as follows:

Figure 11 : TF view of seven dof arm in RViz
The joint state publisher
and robot state publisher
packages are installed along with the ROS desktop's installations.
After creating the robot description of the seven DOF arm, we can discuss how to make a mobile robot with differential wheeled mechanisms.