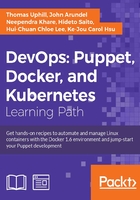
Passing parameters to classes
Sometimes it's very useful to parameterize some aspect of a class. For example, you might need to manage different versions of a gem
package, and rather than making separate classes for each that differ only in the version number, you can pass in the version number as a parameter.
How to do it…
In this example, we'll create a definition that accepts parameters:
- Declare the parameter as a part of the class definition:
class eventmachine($version) { package { 'eventmachine': provider => gem, ensure => $version, } }
- Use the following syntax to include the class on a node:
class { 'eventmachine': version => '1.0.3', }
How it works…
The class definition class eventmachine($version) {
is just like a normal class definition except it specifies that the class takes one parameter: $version
. Inside the class, we've defined a package
resource:
package { 'eventmachine': provider => gem, ensure => $version, }
This is a gem
package, and we're requesting to install version $version
.
Include the class on a node, instead of the usual include
syntax:
include eventmachine
On doing so, there will be a class
statement:
class { 'eventmachine': version => '1.0.3', }
This has the same effect but also sets a value for the parameter as version
.
There's more…
You can specify multiple parameters for a class as:
class mysql($package, $socket, $port) {
Then supply them in the same way:
class { 'mysql': package => 'percona-server-server-5.5', socket => '/var/run/mysqld/mysqld.sock', port => '3306', }
Specifying default values
You can also give default values for some of your parameters. When you include the class without setting a parameter, the default value will be used. For instance, if we created a mysql
class with three parameters, we could provide default values for any or all of the parameters as shown in the code snippet:
class mysql($package, $socket, $port='3306') {
or all:
class mysql( package = percona-server-server-5.5", socket = '/var/run/mysqld/mysqld.sock', port = '3306') {
Defaults allow you to use a default value and override that default where you need it.
Unlike a definition, only one instance of a parameterized class can exist on a node. So where you need to have several different instances of the resource, use define
instead.