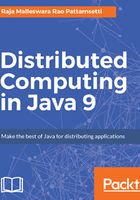
Multicasting
In the previous sections, we learned how to establish a connection and exchange data between one machine and another; this is known as point-to-point communication. But, what if we want to broadcast data over the network to multiple clients? In that case, a subset of the OP protocol that supports multicasting comes in handy.
To broadcast over multicast IP addresses using multicasting, UDP packets are used. Any client listening to a broadcasting IP will receive a broadcasted data packet. A good example of multicasting is broadcasting a video as well as audio of special events over the internet.
Using the java.net.MulticastSocket class, multicasting is possible. This class is an extension of the DatagramSocket class. Any class can create a MulticastSocket object and invoke the joinGroup() method to be able to join the specific multicasted session, as shown in the following code:
MulticastSocket soc = new MulticastSocket(1400);
InetAddress grp = InetAddress.getByName("172.0.114.0");
soc.joinGroup(grp);
After the connection with the multicast session is established, the multicast channel will provide the broadcasted data that can be read by the program:
DatagramPacket pkt;
for (int i = 0; i < 5; i++) {
byte[] buff = new byte[256];
pkt = new DatagramPacket(buff, buff.length);
soc.receive(pkt);
String rcvdString = new String(pkt.getData());
System.out.println("Quote: " + rcvdString);
}
Using the leaveGroup() method, one can disconnect from the session after the broadcast is completed or whenever one is willing to stop the multicast, as follows:
soc.leaveGroup(grp);
soc.close();
Using the thesend() method of MulticastSocket, data can be sent to other listening threads on the multicast channel.
Other examples where multicasting can be used are shareable whiteboards, synchronization tasks between application servers, and load balancing. Since a multicast IP is generally UDP-based, one should be ready for the possibility of losing partial/full data and deal with it gracefully. Also, if a client possesses the capacity to synchronize itself to the ongoing multicast at any time it wants to join, they can choose to join the multicast session at different points of time.