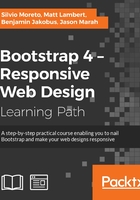
Finishing the web app
To finish the web application example, we just need to create another modal when we click on the Messages link at the navigation bar.
To create it, we will use the same methodology used to create the modal for the Tweet button. So, add the data attributes' markups to the Messages link in .nav.navbar-nav
, as follows:
<ul class="nav navbar-nav">
<li class="active">
<a href="#">
<span class="glyphicon glyphicon-home" aria-hidden="true"></span>
Home
</a>
</li>
<li>
<a href="#">
<span class="badge">5</span>
<span class="glyphicon glyphicon-bell" aria-hidden="true"></span>
Notifications
</a>
</li>
<li>
<a href="#" role="button" data-toggle="modal" data-target="#messages-modal">
<span class="glyphicon glyphicon-envelope" aria-hidden="true"></span>
Messages
</a>
</li>
<li class="visible-xs-inline">
<a href="#">
<span class="glyphicon glyphicon-user" aria-hidden="true"></span>
Profile
</a>
</li>
<li class="visible-xs-inline">
<a href="#">
<span class="glyphicon glyphicon-off" aria-hidden="true"></span>
Logout
</a>
</li>
</ul>
The highlighted code says that this link plays the role
button, toggling a modal identified by the #messages-modal
ID. Create the base of this modal at the end of the HTML code, just after #tweet-modal
:
<div id="messages-modal" class="modal fade" tabindex="-1" role="dialog"> <div class="modal-dialog" role="document"> <div class="modal-content"> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal" aria-label="Close"> <span aria-hidden="true">×</span> </button> <h4 class="modal-title">Dog messages</h4> <button type="button" class="btn btn-primary btn-message">New message</button> </div> <div class="modal-body"> </div> </div> </div> </div>
We made some changes in comparison to #tweet-modal
. Firstly, we removed .modal-footer
from this modal, since we do not need these options in the modal. Like almost the entire framework, Bootstrap allows us to include or exclude elements as per our wishes.
Secondly, we created a new button, New message, in the header, identified by the .btn-message
class. To present the button correctly, create the following CSS style:
#messages-modal .btn-message { position: absolute; right: 3em; top: 0.75em; }
Now let's create the content inside the modal. We will add a list of messages in the modal. Check out the HTML with the content added:
<div class="modal fade" id="messages-modal" tabindex="-1" role="dialog"> <div class="modal-dialog" role="document"> <div class="modal-content"> <div class="modal-header"> <button type="button" class="close" data-dismiss="modal" aria-label="Close"> <span aria-hidden="true">×</span> </button> <h4 class="modal-title">Dog messages</h4> <button type="button" class="btn btn-primary btn-message">New message</button> </div> <div class="modal-body"> <ul class="list-unstyled"> <li> <a href="#"> <img src="imgs/laika.jpg" class="img-circle"> <div class="msg-content"> <h5>Laika <small>@spacesog</small></h5> <p>Hey Jonny, how is down there?</p> </div> </a> </li> <li> <a href="#"> <img src="imgs/doge.jpg" class="img-circle"> <div class="msg-content"> <h5>Doge <small>@dogeoficial </small></h5> <p>Wow! How did I turned in to a meme?</p> </div> </a> </li> <li> <a href="#"> <img src="imgs/cat.jpg" class="img-circle"> <div class="msg-content"> <h5>Cat <small>@crazycat</small></h5> <p>You will never catch me!</p> </div> </a> </li> <li> <a href="#"> <img src="imgs/laika.jpg" class="img-circle"> <div class="msg-content"> <h5>Laika <small>@spacesog</small></h5> <p>I think I saw you in Jupiter! Have you been there recently?</p> </div> </a> </li> </ul> </div> </div> </div> </div>
To finish our job, we just create some style in the CSS in order to display our list correctly:
#messages-modal .modal-body { max-height: 32rem; overflow: auto; } #messages-modal li { padding: 0.75rem; border-bottom: 0.1rem solid #E6E6E6; } #messages-modal li:hover { background-color: #E6E6E6; } #messages-modal li a:hover { text-decoration: none; } #messages-modal li img { max-width: 15%; } #messages-modal .msg-content { display: inline-block; color: #000; } #messages-modal .msg-content h5 { font-size: 1em; font-weight: bold; }
In this CSS, we simply set a maximum height for the modal body, while adding a scroll overflow. For the list and the link, we changed the style for hover and adjusted the font weight, size, and color for display.
Refresh the web browser, click on the Messages link in the navigation bar and see your nice modal, as follows:
