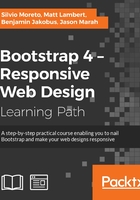
Implementing the main content
Moving on, we will implement the main content in the middle of the page. This content will hold the feeds while allowing new tweets.
We need to create the input to send a new message. To do this, create the following HTML code at the div#main
element:
<div id="main" class="col-sm-12 col-md-6"> <div id="main-card" class="card"> <form id="new-message"> <div class="input-group"> <input type="text" class="form-control" placeholder="What is happening?"> <span class="input-group-addon"> <span class="glyphicon glyphicon-camera" aria-hidden="true"></span> </span> </div> </form> </div> </div>
For that, we created a form, again making use of input groups, icons, and cards. Can you see the ease provided by Bootstrap again? We just placed the elements with the right classes and everything went perfect. The next CSS takes place with some rules regarding the color and padding of the form:
form#new-message { border-radius: 0.4rem 0.4rem 0 0; padding: 1em; border-bottom: 0.1em solid #CEE4F5; background-color: #EBF4FB; } form#new-message .input-group-addon { background-color: #FFF; }
At this point, the result should be as shown in the following screenshot. Next up, we will create the other elements.

Making your feed
We have made cool things so far, but the feed is the core of the page. We will create a nice and friendly feed for our web app.
As usual, let's create the HTML code first. The feed will work inside a stacked list. With that in mind, let's create the first element in the list:
<div id="main" class="col-sm-12 col-md-6"> <div id="main-card" class="card"> <form id="new-message"> <div class="input-group"> <input type="text" class="form-control" placeholder="What is happening?"> <span class="input-group-addon"> <span class="glyphicon glyphicon-camera" aria-hidden="true"></span> </span> </div> </form> <ul id="feed" class="list-unstyled"> <li> <img src="imgs/doge.jpg" class="feed-avatar img-circle"> <div class="feed-post"> <h5>Name <small>@namex - 3h</small></h5> <p> You can't hold a dog down without staying down with him!</p> </div> <div class="action-list"> <a href="#"> <span class="glyphicon glyphicon-share-alt" aria-hidden="true"></span> </a> <a href="#"> <span class="glyphicon glyphicon-refresh " aria-hidden="true"></span> <span class="retweet-count">6</span> </a> <a href="#"> <span class="glyphicon glyphicon-star" aria-hidden="true"></span> </a> </div> </li> </ul> </div> </div>
The highlighted code is the code added for the list. To understand it, we created an element in the list containing the common stuff inside a post, such as an image, a name, text, and options. Add the .img-circle
class to the image in the list to style it using Bootstrap image styles.
With the CSS, we will correctly style the page. For the list and the image, apply the following rules:
ul#feed { margin: 0; } ul#feed li { padding: 1em 1em; } ul#feed .feed-avatar { width: 13%; display: inline-block; vertical-align: top; }
By doing this, you will be correcting the margins and padding while adjusting the size of the image avatar. For the post section, use this CSS:
ul#feed .feed-post { width: 80%; display: inline-block; margin-left: 2%; } ul#feed .feed-post h5 { font-weight: bold; margin-bottom: 0.5rem; } ul#feed .feed-post h5 > small { font-size: 1.2rem; }
Finally, with regard to .action-list
, set the following styles:
ul#feed .action-list { margin-left: 13%; padding-left: 1em; } ul#feed .action-list a { width: 15%; display: inline-block; } ul#feed .action-list a:hover { text-decoration: none; } ul#feed .action-list .retweet-count { padding-left: 0.2em; font-weight: bold; }
Refresh your browser and you will get this result:

Awesome! Note that for the post, we did all the spacing using percentage values. This is also a great option because the page will resize with respect to the user's resolution very smoothly.
We have only one problem now. Add another post and you will see that there is no divisor between the posts. To illustrate this, add a second post in the HTML code:
<ul id="feed" class="list-unstyled"> <li> <img src="imgs/doge.jpg" class="feed-avatar img-circle"> <div class="feed-post"> <h5>Doge <small>@dogeoficial - 3h</small></h5> <p>You can't hold a dog down without staying down with him!</p> </div> <div class="action-list"> <a href="#"> <span class="glyphicon glyphicon-share-alt" aria-hidden="true"></span> </a> <a href="#"> <span class="glyphicon glyphicon-refresh" aria-hidden="true"></span> <span class="retweet-count">6</span> </a> <a href="#"> <span class="glyphicon glyphicon-star" aria-hidden="true"></span> </a> </div> </li> <li> <img src="imgs/laika.jpg" class="feed-avatar img-circle"> <div class="feed-post"> <h5>Laika <small>@spacesog - 4h</small></h5> <p>That's one small step for a dog, one giant leap for giant</p> </div> <div class="action-list"> <a href="#"> <span class="glyphicon glyphicon-share-alt" aria-hidden="true"></span> </a> <a href="#"> <span class="glyphicon glyphicon-refresh" aria-hidden="true"></span> <span class="retweet-count">6</span> </a> <a href="#"> <span class="glyphicon glyphicon-star" aria-hidden="true"></span> </a> </div> </li> </ul>
At the CSS, change it a little to correct the padding and add a border between the items, as follows:
ul#feed li { padding: 1em 1em; border-bottom: 0.1rem solid #e5e5e5; } ul#feed li:last-child { border-bottom: none; }
The output results will be like this:

Doing some pagination
Okay, I know that web applications such as Twitter usually use infinite loading and not pagination, but we need to learn that! Bootstrap offers incredible options for pagination, so let's use some of them right now.
From the start, create the HTML code for the component and insert it just after div.main-card
:
<nav class="text-center"> <ul class="pagination pagination-lg"> <li class="disabled"><a href="#" aria-label="Previous"><span aria-hidden="true">«</span></a></li> <li class="active"><a href="#">1 <span class="sr-only">(current)</span></a></li> <li><a href="#">2</a></li> <li class="disabled"><a href="#">...</a></li> <li><a href="#">3</a></li> <li><a href="#">4</a></li> <li><a href="#" aria-label="Next"><span aria-hidden="true">»</span></a></li> </ul> </nav>
Thus, we must consider a few things here. Firstly, to center the pagination, we used the helper class from Bootstrap, .text-center
. This is because ul.pagination
does apply the display: inline-block
style.
Secondly, we created a <ul>
with the .pagination
class, determined by the framework. We also added the .pagination-lg
class, which is an option of pagination for making it bigger.
Lastly, the .disabled
class is present in two items of the list, the previous link and the ellipsis ...
one. Also, the list on page 1 is marked as active, changing its background color.
Check out the result of adding pagination in this screenshot:
