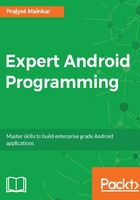
MVC (Model View Controller)
This is one of the most widely used approaches in software development. The MVC consists of three main components:
- Model: The model represents the object in the application. This has the logic of where the data is to be fetched from. This can also have the logic by which the controller can update the view. In Android, the model is mostly represented by object classes.
- View: The view consists of the components that can interact with the user and is responsible for how the model is displayed in the application. In Android, the view is mostly represented by the XML where the layouts can be designed.
- Controller: The controller acts as a mediator between the model and the view. It controls the data flow into the model object and updates the view whenever data changes. In Android, the controller is mostly represented by the activities and fragments.
All of these components interact with each other and perform specific tasks, as shown in the following figure:

It has been noticed that Android is not able to follow the MVC architecture completely, as activity/fragment can act as both the controller and the view, which makes all the code cluttered in one place. Activity/fragment can be used to draw multiple views for a single screen in an app, thus all different data calls and views are populated in the same place. Therefore, to solve this problem, we can use different design patterns or can implement MVC carefully by taking care of conventions and following proper programming guidelines.
The following shows a basic example of how MVC is used in Android :
Model:
public class LocationItem {
String name;
String address;
public LocationItem(String name, String address) {
this.name = name;
this.address = address;
}
public String getName() {
return name;
}
public String getAddress() {
return address;
}
}
View:
<TextView
android:id="@+id/name"
style="@style/HorizontalPlaceTitleTxtStyle"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
Controller:
TextView name = (TextView)findViewById(R.id.name);
name.setText(LocationUtil.getLocationName(list.get(position)));
//Function In LocationUtil(Controller Class)
public class LocationUtil {
public static String getLocationName(LocationItem item) {
return item.getName();
}
}
To explain the MVC pattern components shown in the preceding code; the model here is LocationItem, which holds the name and address of the location. The view is an XML file, which contains a TextView, through which the name of the location can be displayed. The activity, which is the controller, contains a LocationItem. It gets the name of the LocationItem at a specific position in the list and sets it up in the view, which displays it.