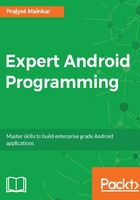
Dependency Inversion Principle
The Dependency Inversion Principle states that:
1. High-level modules should not depend on low-level modules. Both should depend on abstractions.
2. Abstractions should not depend upon details. Details should depend upon abstractions.
The best way to explain this principle is by giving an example. Let's assume we have a worker class that is a low level class and a Manager class that is a high level class. The Manager class contains many complex functionalities which it implements along with the Worker class, so the classes will look something like this:
class Worker { public void work() { // ....working } } class Manager { //--Other Functionality Worker worker; public void setWorker(Worker w) { worker = w; } public void manage() { worker.work(); } }
Here the Manager class has been implemented and is directly associated with the Worker class due to which changes in the Worker class directly affect the Manager class.
If we have to add another class which would be a parent of the Worker class, and the Worker class does similar work to that of the Manager class, it will require lots of changes.
To make it easier to add the Manager class, we will use interfaces:
interface IWorker { public void work(); } class Worker implements IWorker{ public void work() { // ....working } } class SuperWorker implements IWorker{ public void work() { //.... working much more } } class Manager { IWorker worker; public void setWorker(IWorker w) { worker = w; } public void manage() { worker.work(); } }
Now the both the worker and SuperWorker class implement the IWorker, while the Manager class directly uses the IWorker to complete its functionality by which changes to the Worker and SuperWorker do not affect the Manager class.