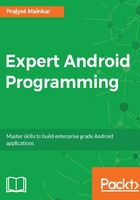
Single Responsibility Principle
The Single Responsibility Principle states that:
A class should have one, and only one, reason to change.
The idea behind this principle is to design a class that has one responsibility or various methods with unique functionality. According to this principle, a method should not do more than one task at a time. Each function must be designated a unique task.
Let's take, for example, the adapter of a recyclerView:
@Override public void onBindViewHolder(final ViewHolder holder, final int position) { PlaceItem item = list.get(position); String name = item.getName() != null ? item.getName() : ""; String description = item.getDescription() != null ? item.getDescription() : ""; String location = item.getAddress() != null ? item.getAddress() : ""; String rating = String.format("%.02f", item.getRating()); String distance = item.getDistance()+"km"; holder.name.setText(name); holder.location.setText(location); holder.description.setText(description); holder.rating.setText(rating); holder.distance.setText(item.getDistance()); Picasso.with(context) .load(R.drawable.im_backdrop) .placeholder(R.drawable.placeholder_200) .error(R.drawable.placeholder_200) .into(holder.image); }
The preceding code is the onBindViewHolder of the adapter of a recyclerView. It does not satisfy the single responsibility principle, as we are formatting the values as we are setting the text to the views. The onBindViewHolder of the recyclerView adapter is mainly responsible for binding the view with the values of its object class.
In the preceding code, the onBindViewHolder is doing more tasks than it should. If we have kept the same format in more than one place and there comes a need to make changes to the format, then we will have to make the changes everywhere and this may result in software logic duplication issues. If we don't update the code in some places, it may cause an error. If the code was a logical change that had been replicated, the entire flow of the app would change. To prevent this, we have to write the code in a way that we don't have to make many changes if the features are changed.
As in the case of the preceding example, we can refactor it by the following:
@Override public void onBindViewHolder(final ViewHolder holder, final int position) { PlaceItem item = list.get(position); holder.name.setText(CommonFunctions.checkNull(item.getName())); holder.description.setText(CommonFunctions.checkNull(item.getDescription())); holder.location.setText(CommonFunctions.checkNull(item.getAddress())); holder.rating.setText(CommonFunctions.formatRating(item.getRating())); holder.distance.setText( CommonFunctions.formatDistance(item.getDistance())); Picasso.with(context) .load(R.drawable.im_backdrop) .placeholder(R.drawable.placeholder_200) .error(R.drawable.placeholder_200) .into(holder.image); }
Now, as can be seen, the code checking and formatting are not done in the onBindViewHolder function but they are being done elsewhere. Due to this, the change of the functionality or format can be done in the common function instead of each instance of the code.