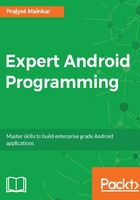
The architecture
The Google Play Service Library contains APIs that provide an interface to the individual Google services. This interface also allows you to obtain authorization from users to gain access to these services using the users' credentials. These services include Google Drive, Play Games and more. Since the library derives updates from Google Play, it does contain APIs that help you resolve any issues at runtime. Sometimes you may have come across apps that prompt you to update Google Play Services before proceeding. The Google Play Service APK runs as a background service in the Android OS and you can interact with the background service though the client library. The service performs the desired action on your behalf.
The table below shows the list of services provided by Google Play Services:

In the app we are working on, we would need Google services such as Google+ Login and Google Maps. To begin with, you must first install the Google Play Services library (revision 15 or higher) for your Android SDK. Also, to include a specific dependency for your app, proceed to add specific services in your app's build Gradle.
The various services provided by Google Play Services could be included in an Android app using a Gradle path in the app's build.gradle. For example:
dependencies {
compile'com.google.android.gms:play-services:10.0.1'
}
Make sure these changes are saved and you click Sync Project with Gradle Files in the toolbar. Since we are using the Google+ Sign in feature, we are required to provide SHA-1 of your signing certificate to create an OAUTH2 client and API key for your app. To generate your SHA-1, please run the following command using the KeyTool utility provided with Java:
keytool -exportcert -list -v \ -alias <your-key-name> -keystore<path-to-production-keystore>
Next, proceed to get the debug certificate fingerprint using the following command:
On Linux/Mac:
keytool -exportcert -list -v \ -alias androiddebugkey -keystore ~/.android/debug.keystore
And on Windows:
keytool -exportcert -list -v \
-alias androiddebugkey -keystore %USERPROFILE%\.android\debug.keystore
The key tool utility should now prompt you to enter a password for the keystore. The default password for the debug keystore is android. Post this, you will able to see the fingerprint displayed onto the terminal.
To know more about signing your Android application, please do check this link:
https://developer.android.com/studio/publish/app-signing.html
Since we are looking to access Google APIs using the users' Google Accounts over HTTP, we have to ensure that the users experience a secure and consistent experience in retrieving an OAuth 2.0 token for your app. To make this possible, you need to register your Android app with the Google Cloud Console by providing your app's package name and the SHA1 fingerprint of the keystore, using which you will sign the release APK.
To register your Android app with Google Cloud Console, follow these simple steps:
- Login to Google Cloud Console: https://console.developers.google.com.
- Click on Create project and enter the project name. In our case, we have named it Zomato Project:

- Click Create. This will create a new project in your Google Developer Console.
Since we have created the project, we will now enable the APIs that are required for the project. To enable the APIs, click on the library tab. Here you will see the list of all APIs that can be added to the app.
Now, let's start by integrating Google+ Login into our app:
- First go to the library tab in the Google developer console.
- Select Google+ API under the Social APIs section and click Enable. Now, the Google + API is enabled for this project.
- Next go to the Credentials. Here we will be creating an OAuth credential for the app so that the user can log in from the Android app. Before we create a new credential, we have to fill in OAuth details of the app:

- After all of these details have been filled, go to the Credentials tab and click on Create credential:

- Then, select the type of application where we are applying the Google+ Login. In our case, it is an Android app, so we would select Android:

- Then you will see an option to add the app's package name and signing-certificate fingerprint.
- Now go to https://developers.google.com/identity/sign-in/android/start and click on GET A CONFIGURATION FILE:

- Here you will see an option to select an app and enter the package name. Fill in the details and click on Choose and configure services:

- At this screen, enable Google Sign In and enter the signing-certificate fingerprint.
- Then click on Generate configuration file:

- You can now download the google-services.json file, which we will be using in the app for services like Google+ Sign In and other services enabled from Google the console:

The google-services.json file is to be placed in the app directory in your project.
Now, add the dependency to your project-level build.gradle: Classpath com.google.gms:google-services:3.0.0.
Also, add this plugin in your app-level build.gradle: Apply plugin com.google.gms.google-services.
Finally, add the dependency into your app-level Gradle: Compile com.google.android.gms:play-services-auth:9.2.1.
We have now completed the setup for Google Play Services for this app. In the next section we will discuss the Android Architecture we would follow, along with discussion on the UI patterns for this app.