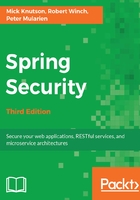
The CalendarUserDetailsService class
Up to this point, we have needed two different representations of users: one for Spring Security to make security decisions, and one for our application to associate our domain objects to. Create a new class named CalendarUserDetailsService that will make Spring Security aware of our CalendarUser object. This will ensure that Spring Security can make decisions based upon our domain model. Create a new file named CalendarUserDetailsService.java, as follows:
//src/main/java/com/packtpub/springsecurity/core/userdetails/
CalendarUserDetailsService.java
// imports and package declaration omitted
@Component
public class CalendarUserDetailsService implements
UserDetailsService {
private final CalendarUserDao calendarUserDao;
@Autowired
public CalendarUserDetailsService(CalendarUserDao
calendarUserDao) {
this.calendarUserDao = calendarUserDao;
}
public UserDetails loadUserByUsername(String username) throws
UsernameNotFoundException {
CalendarUser user = calendarUserDao.findUserByEmail(username);
if (user == null) {
throw new UsernameNotFoundException("Invalid
username/password.");
}
Collection<? extends GrantedAuthority> authorities =
CalendarUserAuthorityUtils.createAuthorities(user);
return new User(user.getEmail(), user.getPassword(),
authorities);
}
}
Within Spring Tool Suite, you can use Shift+Ctrl+O to easily add the missing imports. Alternatively, you can copy the code from the next checkpoint (chapter03.03-calendar).
Here, we utilize CalendarUserDao to obtain CalendarUser by using the email address. We take care not to return a null value; instead, a UsernameNotFoundException exception should be thrown, as returning null breaks the UserDetailsService interface.
We then convert CalendarUser into UserDetails, implemented by the user, as we did in the previous sections.
We now utilize a utility class named CalendarUserAuthorityUtils that we provided in the sample code. This will create GrantedAuthority based on the email address so that we can support users and administrators. If the email starts with admin, the user is treated as ROLE_ADMIN, ROLE_USER. Otherwise, the user is treated as ROLE_USER. Of course, we would not do this in a real application, but it's this simplicity that allows us to focus on this lesson.