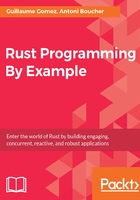
Creating a window
The previous example created a window and drew into it. Now let's see how it did that!
Before going any further, we need to import the SDL2 crate, as follows:
extern crate sdl2;
With this, we now have access to everything it contains.
Now that we've imported sdl2, we need to initialize an SDL context:
let sdl_context = sdl2::init().expect("SDL initialization failed");
Once done, we need to get the video subsystem:
let video_subsystem = sdl_context.video().expect("Couldn't get SDL
video subsystem");
We can now create the window:
let window = video_subsystem.window("Tetris", 800, 600) .position_centered()
.opengl() .build() .expect("Failed to create window");
A few notes on these methods:
- The parameters for the window method are title, width, height
- .position_centered() gets the window in the middle of the screen
- .opengl() makes the SDL use opengl to render
- .build() creates the window by applying all previously received parameters
- .expect panics with the given message if an error occurred
If you try to run this sample of code, it'll display a window and close it super quickly. We now need to add an event loop in order to keep it running (and then to manage user inputs).
At the top of the file, you need to add this:
use sdl2::event::Event; use sdl2::keyboard::Keycode; use std::thread::sleep; use std::time::Duration;
Now let's actually write our event manager. First, we need to get the event handler as follows:
let mut event_pump = sdl_context.event_pump().expect("Failed to
get SDL event pump");
Then, we create an infinite loop to loop over events:
'running: loop { for event in event_pump.poll_iter() { match event { Event::Quit { .. } | Event::KeyDown { keycode: Some(Keycode::Escape), .. } => { break 'running // We "break" the infinite loop. }, _ => {} } } sleep(Duration::new(0, 1_000_000_000u32 / 60)); }
To go back on these two lines:
'running: loop { break 'running
loop is a keyword that allows creating an infinite loop in Rust. An interesting feature though is that you can add a label to your loops (so, while and for as well). In this case, we added the label running to the main loop. The point is to be able to break directly an upper loop without having to set a variable.
Now, if we receive a quit event (pressing the cross of the window) or if you press the Esc key, the program quits.
Now you can run this code and you'll have a window.