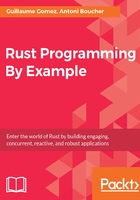
Associated types
We can also have types in a trait that need to be specified. For instance, let's implement the Add trait from the standard library on our Point type that we declared earlier, which allows us to use the + operator on our own types:
use std::ops::Add; impl Add<Point> for Point { type Output = Point; fn add(self, point: Point) -> Self::Output { Point { x: self.x + point.x, y: self.y + point.y, } } }
The first line is to import the Add trait from the standard library so that we can implement it on our type. Here we specify that the associated Output type is Point. Associated types are most useful for return types. Here, the Output of the add() method is the associated Self::Output type.
Now, we can use the + operator on Points:
let p1 = Point { x: 1, y: 2 }; let p2 = Point { x: 3, y: 4 }; let p3 = p1 + p2;
Having to specify the output parameter with an associated type (instead of setting it to Self) gives us more flexibility. For instance, we could implement the scalar product for the * operator, which takes two Points and returns a number.
You can find all the operators that can be overloaded on this page, at https://doc.rust-lang.org/stable/std/ops/index.html.
Since Rust 1.20, Rust also supports associated constants in addition to associated types.