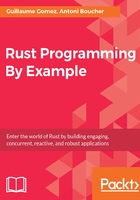
上QQ阅读APP看书,第一时间看更新
Constructors
Rust does not provide constructors, but a common idiom is to create a new() static method, also called an associated function:
impl Point { fn new(x: i32, y: i32) -> Self { Self { x: x, y: y } } }
The difference with a normal method is that it does not take &self (or one of its variations) as a parameter.
Self is the type of the self value; we could have used Point instead of Self.
When the field name is the same as the value assigned, it is possible to omit the value, as a shorthand:
fn new(x: i32, y: i32) -> Self { Self { x, y } }
When we create an instance of Point with the call to its constructor (let point = Point::new();), this will allocate the value on the stack.
We can provide multiple constructors:
impl Point { fn origin() -> Self { Point { x: 0, y: 0 } } }