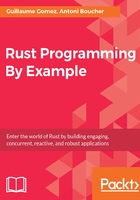
上QQ阅读APP看书,第一时间看更新
Copy types
Some types are not moved when we assigned a value of these types to another variable. This is the case for basic types such as integers. For instance, the following code is perfectly valid:
let num1 = 42; let num2 = num1; println!("{}", num1);
We can still use num1 even thought we assigned it to num2. This is because the basic types implement a special marker: Copy. Copy types are copied instead of moved.
We can make our own types Copy by adding derive to them:
#[derive(Clone, Copy)] struct Point { x: i32, y: i32, }
Since Copy requires Clone, we also implement the latter for our Point type. We cannot derive Copy for a type containing a value that does not implement Copy. Now, we can use a Point without having to bother with references:
fn print_point(point: Point) { println!("x: {}, y: {}", point.x, point.y); } let p1 = Point { x: 1, y: 2 }; let p2 = p1; print_point(p1); println!("{}", p1.x);