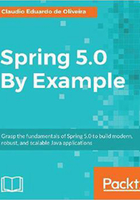
上QQ阅读APP看书,第一时间看更新
Creating the Category Service
The CategoryService objects is a singleton object because it is an AngularJS service. The service will interact with our CMS APIs powered by the Spring Boot application.
We will use the $http service. It makes the HTTP communications easier.
Let's write the CategoryService:
(function (angular) {
'use strict';
/* Services */
</span> angular.module('cms.modules.category.services', []).
service('CategoryService', ['$http',
function ($http) {
var serviceAddress = 'http://localhost:8080';
var urlCollections = serviceAddress + '/api/category';
var urlBase = serviceAddress + '/api/category/';
this.find = function () {
return $http.get(urlCollections);
};
this.findOne = function (id) {
return $http.get(urlBase + id);
};
this.create = function (data) {
return $http.post(urlBase, data);
};
this.update = function (data) {
return $http.put(urlBase + '/id/' + data._id, data);
};
this.remove = function (data) {
return $http.delete(urlBase + '/id/' + data._id, data);
};
}
]);
})(angular);
Well done, now we have implemented the CategoryService.
The .service function is a constructor to create a service instance, the angular acts under the hood. There is an injection on a constructor, for the service we need an $http service to make HTTP calls against our APIs. There are a couple of HTTP methods here. Pay attention to the correct method to keep the HTTP semantics.