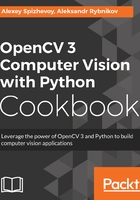
上QQ阅读APP看书,第一时间看更新
How to do it...
For this recipe, we need the following steps to be executed:
- Load an image and print its original size:
img = cv2.imread('../data/Lena.png')
print('original image shape:', img.shape)
- OpenCV offers several ways of using the cv2.resize function. We can set the target size (width, height) in pixels as the second parameter:
width, height = 128, 256
resized_img = cv2.resize(img, (width, height))
print('resized to 128x256 image shape:', resized_img.shape)
- Resize by setting multipliers of the image's original width and height:
w_mult, h_mult = 0.25, 0.5
resized_img = cv2.resize(img, (0, 0), resized_img, w_mult, h_mult)
print('image shape:', resized_img.shape)
- Resize using nearest-neighbor interpolation instead of the default one:
w_mult, h_mult = 2, 4
resized_img = cv2.resize(img, (0, 0), resized_img, w_mult, h_mult, cv2.INTER_NEAREST)
print('half sized image shape:', resized_img.shape)
- Reflect the image along its horizontal x-axis. To do this, we should pass 0 as the last argument of the cv2.flip function:
img_flip_along_x = cv2.flip(img, 0)
- Of course, it's possible to flip the image along its vertical y-axis—just pass any value greater than 0:
img_flip_along_y = cv2.flip(img, 1)
- We can flip both x and y simultaneously by passing any negative value to the function:
img_flipped_xy = cv2.flip(img, -1)