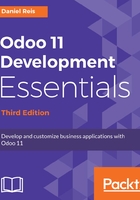
Extending existing models
New models are defined through Python classes. Extending models is also done through Python classes, with help from an Odoo-specific inheritance mechanism, the _inherit class attribute. This _inherit class attribute identifies the model to be extended. The new class inherits all the features of the parent Odoo model, and we only need to declare the modifications to introduce.
In our todo.task model, we have a many-to-many field for the team of people (Partners) that will collaborate with us on that Task. We will now add to the Partners model the inverse of that relation, so that we can see, for each Partner, all the Tasks they are involved in.
The coding style guidelines recommend having a Python file for each model. So we will add a models/res_partner_model.py file to implement our extensions on Partners.
Edit the models/__init__.py file to also import this new code file. The content should look like this:
from . import todo_task_model
from . import res_partner_model
Now create the models/res_partner_model.py with the following code:
from odoo import fields, models class ResPartner(models.Model): _inherit = 'res.partner' todo_ids= fields.Many2many( 'todo.task', string="To-do Teams")
We used the _inherit class attribute to declare the Model to extend. Notice that we didn't use any other class attributes, not even the _name. This is not needed, unless we want to make changes to any of them.
You can think of this as getting a reference to a Model definition living in a central registry, and making in-place changes to it. These can be adding fields, modifying existing fields, modifying model class attributes, or even methods with business logic.
In our case, we add a new field, todo_ids, with the inverse of the relation between Tasks and Partners, defined in the To-Do Task model. There are some details here that are being automatically handled by the ORM, but they are not relevant for us right now. They are addressed in more detail in Chapter 4, Models - Structure the Application Data.
Extending existing fields follows a similar logic: we only need to use the attributes to be modified. All omitted attributes will keep the original value.
To have the new Model fields added to the database tables, we should now perform a module upgrade. If everything goes as expected, the newly added field should be seen when inspecting the res.partner model, in the Technical | Database Structure | Models menu option.