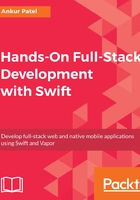
上QQ阅读APP看书,第一时间看更新
Refactoring to share code
Perform the following steps:
- Create a new empty Swift file in our Controllers folder and call it BaseTableViewController.swift.
- Inside of our BaseTableViewController.swift file, create a new class that inherits from the UITableViewController and has a requestInput method:
import UIKit
class BaseTableViewController: UITableViewController {
func requestInput(title: String, message: String, handler:
@escaping (String) -> ()) {
let alert = UIAlertController(title: title,
message: message,
preferredStyle: .alert)
alert.addTextField(configurationHandler: nil)
alert.addAction(UIAlertAction(title: "Cancel", style: .cancel,
handler: nil))
alert.addAction(UIAlertAction(title: "Add", style: .default,
handler: { (_) in
if let listName = alert.textFields?[0].text {
handler(listName)
}
}))
self.present(alert, animated: true, completion: nil)
}
}
- We've created a BaseTableViewController class so that we can subclass our View Controllers from BaseTableViewController and share the requestInput method, which will do all of the heavy lifting of creating an UIAlertController and presenting to get user input. All we have to do now is call this method in our ItemTableViewController and pass it a title, a message, and a handler, which will return us the text that was entered as input by the user in our handler.
- Switch the files and in our ItemTableViewController and change the class inheritance from UITableViewController to BaseTableViewController:
class ItemTableViewController: BaseTableViewController {
- Update the didSelectAdd method to use the inherited requestInput method by updating the body of the method to this:
@IBAction func didSelectAdd(_ sender: UIBarButtonItem) {
requestInput(title: "New shopping list item",
message: "Enter item to add to the shopping list:",
handler: { (itemName) in
let itemCount = self.items.count;
let item = Item(name: itemName)
self.items.append(item)
self.tableView.insertRows(at: [IndexPath(row: itemCount, section: 0)], with: .top)
})
}
Now run the app and it should work as before, but we've refactored it to be able to share the code to get input from the user and inherit any new View Controllers from BaseTableViewController that require an easy way to get input from users using UIAlertView.