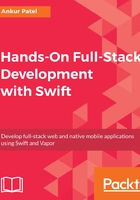
上QQ阅读APP看书,第一时间看更新
Table View Controller
To get our app to do something interesting, we will now need to write some code. We begin with our ItemTableViewController. Opening the file, you will see there is some template code already and some code that has been commented. We will fill out these code blocks one by one to understand what each of these methods does. In our controller, we need to do the following:
- Define a controller instance property called item, which will be an array of Item objects. This is the same Item class we created earlier in this chapter. We will set items to default to the fake items that we will get from the fake method defined on Item class that you created in the earlier exercise:
class ItemTableViewController: UITableViewController {
var items: [Item] = Item.fake(10)
- In our viewDidLoad method, which we touched upon earlier in the View Controller life cycle, we will add the following code. In this code block, we are first calling viewDidLoad, defined in our parent class using super. Then we set the title that is shown in the navigation bar. Finally, starting in iOS 11, the navigation bar can have a large title which we set to true:
super.viewDidLoad()
self.title = "Shopping List Items"
self.navigationController?.navigationBar.prefersLargeTitles = true
- We need to tell the app how many sections to render. By default, the numberOfSections method returns 0 but we need to change this by removing the warning and change the return value to 1. Sections are a way to split apart a tableView, but in our app we just need one to list all of the items:
override func numberOfSections(in tableView: UITableView) -> Int {
return 1
}
- The next method we need to update is tableView(_:numberOfRowsInSection:). This method is called for each section in your tableView and you are passed the section number to figure out how many rows you want to display in each section. In our app, we will have one row for each item, so we need to return the size of our items array:
override func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return items.count
}
- The next method we need to fill out is tableView(_:cellForRowAt:). This method is called with an indexPath that contains the section and row in that section. Using this, we need to return a cell view that is displayed in that section and row. In iOS, there is a convention to reuse cells instead of creating new ones for each row, as there can be a lot or an infinite number or rows that can be generated and, hence, a cell is reused and the subviews in the cells are rendered with the data for cell of that specific row. In our case, we will get the item for the row it is being requested for, then get a reusable cell, and set the value on the subviews of that reusable cell:
let cell = tableView.dequeueReusableCell(withIdentifier: "ItemCell", for: indexPath)
let item = items[indexPath.row]
cell.textLabel?.text = item.name
if item.isChecked {
cell.accessoryType = .checkmark
} else {
cell.accessoryType = .none
}
return cell
- In the previous code block, we get the cell from the table view using tableView.dequeueReusableCell(withIdentifier: "ItemCell", for: indexPath). We need to define ItemCell in our storyboard inside the Table View for it to return the cell. We do this by going to our Main.storyboard and selecting our Table View from the Root View Controller and selecting the Table View Cell. Then, we go to the attributes Inspector on the right and add ItemCell to the Identifier field, which will change the name of the cell to ItemCell in the storyboard. Finally, change the Style to Basic from the Attribute Inspector for this cell:
- Let's run our app and see how it looks. Hit the play button or press on command + R to run the app:

Much better. Now we can see the list of items on our Shopping List. Let's now add the ability to add new items to our list.