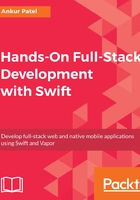
Blueprinting the Shopping List Item model
Now that we understand the files and folders in our projects a little bit, let's start writing some code. We will begin by writing code for our first model, the Shopping List Item. To do so, perform the following steps:
- Create a new group called Models under the ShoppingList folder in your project.
- Then right-click and click on New File... under the Models folder and select a Swift file from the iOS template. Call this new file Item.swift and click Create.
- Copy the following code into the Item.swift file:
import UIKit
class Item {
var name: String
var isChecked: Bool
init(name: String, isChecked: Bool = false) {
self.name = name
self.isChecked = isChecked
}
}
Let's go over the code in more detail:
We define a class called Item which will serve as a blueprint for our Shopping List Items:
class Item {
We then define two properties to store a name for the item and the state of the item on whether it is checked or unchecked. These two properties are called name and isChecked and their types are String and Bool, respectively:
var name: String
var isChecked: Bool
Lastly, we define a construction that takes in one require argument, which is name, and the second optional argument, which is the property value for isChecked that defaults to false:
init(name: String, isChecked: Bool = false) {
self.name = name
self.isChecked = isChecked
}
That is our model to store the data of our Shopping List Item. We will look at how we can create an instance of our model in the controller and then show them in the table view. Before we do, it's time for a short exercise. The solution of this exercise will be used later in the app to generate fake items to show some data in the app when it launches, instead of seeing an empty table view on launch.