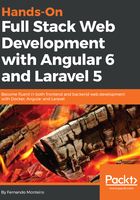
Creating an Angular service
Angular services are used to handle data; it can be internal data (from one component to another) or something external, such as communicating with an API endpoint. Almost all frontend applications with JavaScript frameworks use this technique. In Angular, we call this a service, and we use some modules built into the Angular framework to complete the task: HttpClient and HttpClientModule.
Let's look at how the Angular CLI can help us:
- Open VS Code, and, inside the integrated Terminal, type the following command:
ng g service beers/beers
The previous command will generate two new files in the beers folder:
beers.service.spec.ts and beers.service.ts.
- Add the newly created Service as a dependency provider to beers.module.ts. Open src/app/beers/beers.module.ts and add the following lines:
import { BeersService } from './beers.service';
@NgModule({
providers: [BeersService]
})
In VS Code, we have import module support, so, when you start to type the module's name, you will see the following help screen:

The final beers.module.ts code will look as follows:
import { NgModule } from '@angular/core';
import { CommonModule } from '@angular/common';
import { BeersComponent } from './beers.component';
import { BeersService } from './beers.service';
@NgModule({
imports: [
CommonModule
],
declarations: [BeersComponent],
providers: [BeersService
]
})
export class BeersModule { }
Now, it's time to connect with an API using a service. To get as close to a real application as possible, we'll use a public API in this example. In the next steps, we will effectively create our service and add data binding to our template.
For this example, we will use the free https://punkapi.com/ API:
- Open beers.service.ts and replace the code with the following lines:
import { Injectable } from '@angular/core';
import { HttpClient, HttpHeaders, HttpErrorResponse } from '@angular/common/http';
import { Observable } from 'rxjs/Observable';
import 'rxjs/add/observable/throw';
import { catchError } from 'rxjs/operators';
@Injectable()
export class BeersService {
private url = 'https://api.punkapi.com/v2/beers?';
constructor(private http: HttpClient) { }
/**
* @param {page} {perpage} Are Page number and items per page
*
* @example
* service.get(1, 10) Return Page 1 with 10 Items
*
* @returns List of beers
*/
get(page: number, per_page: number) {
return this.http.get(this.url + 'page=' + page +
'&per_page=' + per_page)
.pipe(catchError(error => this.handleError(error)));
}
private handleError(error: HttpErrorResponse) {
return Observable.throw(error);
}
}
Now, we need to tell the component that we need to use this service to load the data and transmit it to our template.
- Open src/app/beers/beers.component.ts and replace the code with the following:
import { Component, OnInit } from '@angular/core';
import { BeersService } from './beers.service';
@Component({
selector: 'app-beers',
templateUrl: './beers.component.html',
styleUrls: ['./beers.component.css']
})
export class BeersComponent implements OnInit {
public beersList: any [];
public requestError: any;
constructor(private beers: BeersService) { }
ngOnInit() {
this.getBeers();
}
/**
* Get beers, page = 1, per_page= 10
*/
public getBeers () {
return this.beers.get(1, 20).subscribe(
response => this.handleResponse(response),
error => this.handleError(error)
);
}
/**
* Handling response
*/
protected handleResponse (response: any) {
this.requestError = null;
return this.beersList = response;
}
/**
* Handling error
*/
protected handleError (error: any) {
return this.requestError = error;
}
}