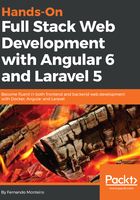
Declaring an interface
Interfaces are our allies when we use TypeScript, since they do not exist in pure JavaScript. They are an efficient way of grouping and typing variables, ensuring that they are always together, maintaining consistent code.
Let's look at a practical way to declare and use an interface:
- In your text editor, create a new file called band-interface.ts, and add the following code:
interface Band {
name: string,
total_members: number
}
To use it, assign the interface to a function type, as in the following example.
- Add the following code right after the interface code, in the band-interface.ts file:
interface Band {
name: string,
total_members: number
}
function unknowBand(band: Band): void {
console.log("This band: " + band.name + ", has: " + band.total_members + " members");
}
Note that here, we are using the Band interface to type our function parameter. So, when we try to use it, we need to keep the same structure in new objects, as in the following example:
// create a band object with the same properties from Band interface:
let newband = {
name: "Black Sabbath",
total_members: 4
}
console.log(unknowBand(newband));
tsc band-interface.ts and the band-interface.js node in your Terminal.
So, if you follow the preceding tip, you will see the same result in your Terminal window:
This band: Black Sabbath, has: 4 members
As you can see, the interfaces in TypeScript are incredible; we can do a lot of things with them. Throughout the course of this book, we will look at some more examples of using interfaces in real web applications.