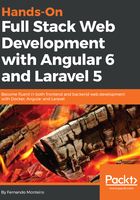
上QQ阅读APP看书,第一时间看更新
Creating a tuple
A tuple is like an organized typed array. Let's create one to see how it works:
- Inside the chapter-02 folder, create a file called tuple.ts, and add the following code:
const organizedArray: [number, string, boolean] = [0, 'text',
false];
let myArray: [number, string, boolean];
myArray = ['text', 0, false]
console.log(myArray);
The preceding code looks fine for JavaScript, but in TypeScript, we must respect the variable type; here, we are trying to pass a string where we must pass a number.
- In your Terminal, type the following command:
tsc tuple.ts
You will see the following error message:
tuple.ts(4,1): error TS2322: Type '[string, number, false]' is not assignable to type '[number, string, boolean]'.
Type 'string' is not assignable to type 'number'.
In VS Code, you will see the error message before you compile your file. This is a very helpful feature.
When we fix it with the right order (myArray = [0, 'text', false]), the error message disappears.
It's also possible to create a tuple type and use it to assign a variable, as we can see in the next example.
- Go back to your Terminal and add the following code to the tuple.ts file:
// using tuple as Type
type Tuple = [number, string, boolean];
let myTuple: Tuple;
myTuple = [0, 'text', false];
console.log(myTuple);
At this point, you may be wondering why the previous examples have a console.log output.
With the help of Node.js, which we installed previously, we can run the examples and view the output of the console.log() function.
- Inside the Terminal, type the following command:
node tuple.js
Note that you will need to run the JavaScript version, as in the previous example. If you try to run the TypeScript file directly, you will probably receive an error message.