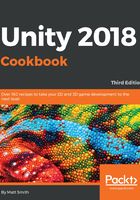
上QQ阅读APP看书,第一时间看更新
How to do it...
To schedule a sound to play after a given delay, do the following:
- Create a new 3D project and import the provided sound clip files.
- In the Inspector, set the Background of the Main Camera to black.
- Set the Main Camera Transform Position to (224, 50, -200).
- Set the Main Camera Camera component to have these settings: Projection = Perspective, Field of View 60, and Clipping Planes 0.3 - 300.
- Add DirectionalLight to the scene.
- Add a new empty GameObject named visualizer to the scene. Add an AudioSource component to this GameObject, and set its AudioClip to one of the 140 bmp loops provided.
- Create a C# script class, SpectrumCubes, in a new folder, _Scripts, containing the following code, and add an instance as a scripted component to the visualizer GameObject:
using UnityEngine; public class SpectrumCubes : MonoBehaviour { const int NUM_SAMPLES = 512; public Color displayColor; public float multiplier = 5000; public float startY; public float maxHeight = 50; private AudioSource audioSource; private float[] spectrum = new float[NUM_SAMPLES]; private GameObject[] cubes = new GameObject[NUM_SAMPLES]; void Awake() { audioSource = GetComponent<AudioSource>(); CreateCubes(); } void Update() { audioSource.GetSpectrumData(spectrum, 0, FFTWindow.BlackmanHarris); UpdateCubeHeights(); } private void UpdateCubeHeights() { for (int i = 0; i < NUM_SAMPLES; i++) { Vector3 oldScale = cubes[i].transform.localScale; Vector3 scaler = new Vector3(oldScale.x, HeightFromSample(spectrum[i]), oldScale.z); cubes[i].transform.localScale = scaler; Vector3 oldPosition = cubes[i].transform.position; float newY = startY + cubes[i].transform.localScale.y / 2; Vector3 newPosition = new Vector3(oldPosition.x, newY, oldPosition.z); cubes[i].transform.position = newPosition; } } private float HeightFromSample(float sample) { float height = 2 + (sample * multiplier); return Mathf.Clamp(height, 0, maxHeight); } private void CreateCubes() { for (int i = 0; i < NUM_SAMPLES; i++) { GameObject cube = GameObject.CreatePrimitive(PrimitiveType.Cube); cube.transform.parent = transform; cube.name = "SampleCube" + i; Renderer cubeRenderer = cube.GetComponent<Renderer>(); cubeRenderer.material = new Material(Shader.Find("Specular")); cubeRenderer.sharedMaterial.color = displayColor; float x = 0.9f * i; float y = startY; float z = 0; cube.transform.position = new Vector3(x, y, z); cubes[i] = cube; } } }
- With the visualizer GameObject selected in the Hierarchy, click to choose a visualization color from the Inspector Display Color public variable for the SpectrumCubes (Script) component.
- Run the scene – you should see the cubes jump up and down, presenting a Run-Time visualization of the sound data spectrum for the playing sound.