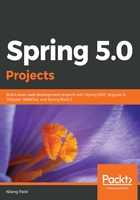
Reactor lifecycle methods
Reactor provides lifecycle methods to capture various events happening in publisher-subscriber communication. Those lifecycle methods are aligned with Reactive Streams specification. Reactor lifecycle methods can be used to hook custom implementation for a given event. Let's understand how that works with the following code:
public class ReactorLifecycleMethods {
public static void main(String[] args) {
List<String> designationList = Arrays.asList(
"Jr Consultant","Associate Consultant","Consultant",
"Sr Consultant","Principal Consultant");
Flux<String> designationFlux = Flux.fromIterable(designationList);
designationFlux.doOnComplete(
() -> System.out.println("Operation Completed ..!!"))
.doOnNext(
value -> System.out.println("value in onNext() ->"+value))
.doOnSubscribe(subscription -> {
System.out.println("Fetching the values ...!!");
for(int index=0; index<6;index++) {
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
subscription.request(1);
}
})
.doOnError(
throwable-> {
System.out.println("Opps, Something went wrong ..!! "
+throwable.getMessage());
})
.doFinally(
(signalType->
System.out.println("Shutting down the operation, Bye ..!! "
+signalType.name())))
.subscribe();
}
We are creating the Flux object with data from a list and then calling various lifecycle methods, like doOnComplete(), doOnNext(), doOnSubscribe(), doOnError(), and doOnTerminate() in a chain. Finally, we call the subscribe() method, which does not consume the events, but all lifecycle methods will be executed as appropriate events are triggered.
This is similar to the custom subscriber implementation in the Custom subscribers section. You will see a similar output. The details of these lifecycle methods are as follows:
- doOnComplete(): Once all the data is received by the Subscriber, this method will be called.
- doOnNext(): This method will listen to the value event coming from the producer.
- doOnSubscribe(): Used to plug Subscription. It can control the backpressure by defining how many more elements are required with a subscription.request() call.
- doOnError(): If any error occurs, this method will be executed.
- doOnTerminate(): Once the operation is completed, either successfully or with error, this method will be called. It will not be considered on a manual cancellation event.
- doOnEach(): As the name suggests, it will be called for all Publisher events raised during stream processing.
- doFinally(): This will be called on stream closures due to error, cancellation, or successful completion of events.