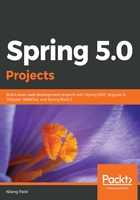
Cold Observable
When the Observable itself creates the procedure or, say, Observable produces the data stream itself, it is said to be cold Observable. Generally Observable is lazy in nature, meaning it only emits the data when any Observer subscribes to it. Cold Observable always starts a fresh execution for each subscriber.
In other words, cold Observable emits separate data/event streams for individual Observers. All examples we have seen so far were of a cold Observable type, where we have created a data stream with the just() or create() method. Let's see how cold Observable works for more than one Observer subscribed, with the following example.
public class RxJavaColdObservable {
public static void main(String[] args) {
Observable<String> source =
Observable.just("One","Two","Three","Four","Five");
//first observer
source.filter(data->data.contains("o"))
.subscribe(data -> System.out.println("Observer 1 Received:" + data));
//second observer
source.subscribe(data -> System.out.println("Observer 2 Received:" + data));
}
}
In this code, the data is created by Observable itself so it is called cold Observable. We have subscribed two different Observers. When you run this code, you will get an output as follows:

Cold Observable provides a separate data stream for each Observer so when we applied the filter for first Observer there is no effect in the second Observer. Also if there are more than one Observer, then Observable will emit the sequence of data to all observers one by one.