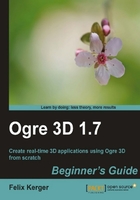
We will use the code we created before and modify it a bit to see how a spotlight works:
- Delete the code where we created the light and insert the following code to create a new scene node. Be careful not to delete the part of the code we used to create
LigthEnt
and then add the following code:Ogre::SceneNode* node2 = node->createChildSceneNode("node2"); node2->setPosition(0,100,0);
- Again, create a light, but now set the type to spotlight:
Ogre::Light* light = mSceneMgr->createLight("Light1"); light->setType(Ogre::Light::LT_SPOTLIGHT);
- Now set some parameters; we will discuss their meanings later:
light->setDirection(Ogre::Vector3(1,-1,0)); light->setSpotlightInnerAngle(Ogre::Degree(5.0f)); light->setSpotlightOuterAngle(Ogre::Degree(45.0f)); light->setSpotlightFalloff(0.0f);
- Set the light color and add the light to the newly created scene node:
light->setDiffuseColour(Ogre::ColourValue(0.0f,1.0f,0.0f)); node2->attachObject(light);
- Compile and run the application; it should look like this:
We created a spotlight in the same manner we created a point light; we just used some different parameters for the light.
Step 1 created another scene node to be used later. Step 2 created the light as we did before; we just used a different light type – this time Ogre::Light::LT_SPOTLIGHT
– to get a spotlight. Step 3 is the really interesting one; there we set different parameters for the spotlight.
Spotlights are just like flashlights in their effect. They have a position where they are and a direction in which they illuminate the scene. This direction was the first thing we set after creating the light. The direction simply defines in which direction the spotlight is pointed. The next two parameters we set were the inner and the outer angles of the spotlight. The inner part of the spotlight illuminates the area with the complete power of the light source's color. The outer part of the cone uses less power to light the illuminated objects. This is done to emulate the effects of a real flashlight. A real flashlight also has an inner part and an outer part that illuminate the area lesser then the center of the spotlight. The inner and outer angles we set define how big the inner and the outer part should be. After setting the angles, we set a falloff
parameter. This falloff
parameter describes how much power the light loses when illuminating the outer part of the light cone. The farther away a point to be illuminated is from the inner cone, the more the falloff
affects the point. If a point is outside the outer cone, then it isn't illuminated by the spotlight.

We set the falloff
to zero. In theory, we should see a perfect light circle on the plane, but it is rather blurry and deformed. The reason for this is that the lighting that we use at the moment uses the triangle points of the plane to calculate and apply the illumination. When creating the plane, we told Ogre 3D that the plane should be created with 20 X 20 segments. This is a rather low resolution for such a big plane and means the light cannot be calculated accurately enough, because there are too few points to apply in an area to make a smooth circle. So to get a better quality render, we have to increase the segments of the plane. Let's say we increase the segments from 20 to 200. The plane creation code looks like this after the increase:
Ogre::MeshManager::getSingleton().createPlane("plane", ResourceGroupManager::DEFAULT_RESOURCE_GROUP_NAME, plane, 1500,1500,200,200,true,1,5,5,Vector3::UNIT_Z);
Now when recompiling and restarting the application, we get a nice round circle of light from our spotlight.

The circle still isn't perfect; if needed, we could increase the segments of the plane even further to make it perfect. There are different lighting techniques which give better results with a low-resolution plane, but they are rather complex and would complicate things now. But even with the complex lighting techniques, the basics are the same and we can change our lighting scheme later using the same lights we created here.
In step 4, we saw another way to describe a color in Ogre 3D. Previously, we used three values, (r,g,b), to set the diffuse color of our light. Here we used Ogre::ColourValue
(r,g,b), which is basically the same but encapsulated as a class with some additional functions and thus it makes the intention of the parameter clearer.
Describe the difference between a point light and a spotlight in a few words.
Create a second spotlight that is at a different position as compared to the first spotlight. Give this spotlight a red color and position it in such a way that the circles of both spotlights overlap each other a bit. You should see that the color is mixing in the area where the green and red light overlap.
