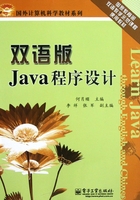
3.9 Java’s Floating Point Types
3.9.1 Primitive Floating Point Types
Java supplies two different primitive floating point data types.Table 3.2 lists characteristics of two floating point types.
Java提供了两种浮点数据类型,其特征如表3.2所示。
Table 3.2 Java’s primitive floating point types.
The minimum and maximum values of the types are declared as manifest constant values in the corresponding Number class.For example the minimum and maximum double values are declared as java.lang.Double.MIN_VALUE and java.lang.Double.MAX_VALUE.
3.9.2 Integer Operators
The operators for the double data types are listed with examples. The operators for the float type are essentially identical.Table 3.3 lists relative operators of float data types.
Table3.3 The operators for float data type
3.9.3 Input and Output of Floating Point Values
The considerations(事项)for the input and output of floating point values are essentially identical to those for integer data types.
3.9.4 Casting of Floating Point to and from Integer Values, and Floating Point Literals
Casting a floating point value from a float to a double representation(表示法)is never dangerous, as it can be guaranteed that a double can always store the value accurately.
For example the following can never result in a dangerous operation and upon completion both variables will have the same value.
float floatVariable = someFloatValue; double doubleVariable; doubleVariable = floatVariable;
Although this is acceptable by Java it is regarded as good style to always indicate that the developer is aware that a type conversion is taking place by explicitly indicating a cast in the assignment.
doubleVariable = (double) floatVariable;
Likewise any integer value can be automatically(自动地) or explicitly(显式地) cast to either floating point type without any danger, as the floating point variable will always be able to represent it. However the converse(相反的) conversion, from any floating point type to any integer type, can be accomplished by an explicit cast but will result in an unpredictable(不可预知的) value unless the floating point value is within the limits of the integer type’s range. This can be guarded against as shown in the following fragment.
if (((long) doubleVariable) >= java.lang.Long.MIN_VALUE && ((long) doubleVariable) <= java.lang.Long.MAX_VALUE ) { longVariable = (long) doubleVariable; } else { // Value cannot be converted } // End if
Floating point literals can be expressed in conventional ( e.g. 345.678) or exponent notation (e.g. 3.45678e+02), but both mantissa and exponent must always be expressed as denary (base 10) values. By default such literals are always assumed to be of type double. Should it ever be required a double value can be indicated by appending a D and a float by appending a F, e.g. 345.678D or 345.678F.
浮点型数字可以用传统的符号和指数符号来表示。但它们的尾数和指数必须用十进制数表示。这些数字默认为double类型。为了明确表示double值要附加一个D,而float值要附加一个F,如345.678D,345.678F。
3.9.5 Floating Point Operations in the Standard Packages
A random float or double value between 0.0 and 1.0 can be obtained from the class-wide nextFloat( ) or nextDouble( ) actions in the java.util.Random class. The random number package is automatically seeded from the system clock which will ensure that a different sequence of random numbers is obtained every time an instance is constructed.
The java.lang.Math class supplies a class-wide random( ) action which can sometimes be more conveniently used to obtain a double value between 0.0 and 1.0. Other actions and class wide constants in java.lang.Math. Table 3.4 lists functions in java.lang.Math.
Table 3.4 Java.lang.Math
3.9.6 The Float Class
In addition to the primitive floating point types Java also supplies two classes which implement the double and float types as objects, the major reason for this is to allow them to be used with Java’s utility classes. For example if you wanted to store double values in a hash table then only Double instances could be used, not primitive double variables.
The two packages are java.lang.Number.Double and java.lang.Number.Float., the Double class is essentially identical. Table 3.5 lists functions in class java.lang.Number.Float.
Table 3.5 java.lang.Number.Float